Hello developers in this tutorial we will discuss about how to send push notification using google firebase console in laravel 10
Table of Contents
1:Basic setup of google firebase console
Google firebase console provide us service to send push notification and its free to use so below are the steps for google console
1:Go to Google Firebase Console and click on create project
2:Enter project name and click on continue
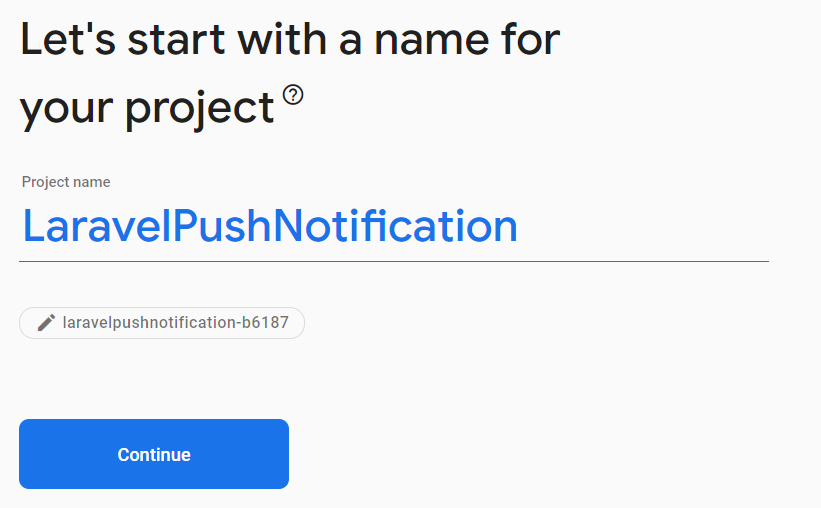
3:And click continue then select your country and click on continue
4:Select Web option
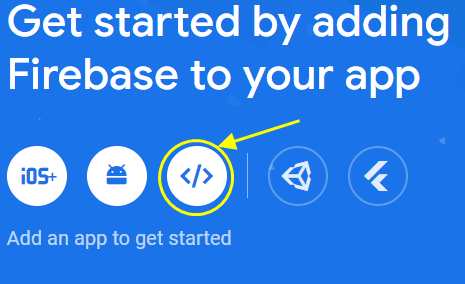
5:Enter App nickname and click on register and the continue to console.
6: On left side click on project settings
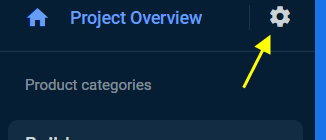
7:In General setting you will see your application credentials of console
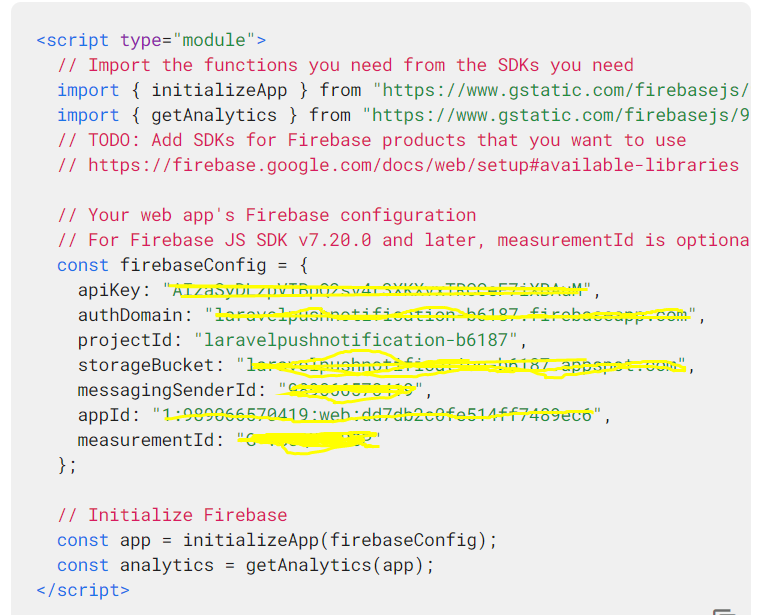
8:Then go to Cloud messaging and then if you see disable api , then enable it first by clicking on 3 dots.

9:After enabling come to previous page and reload it , you will able to see a server key
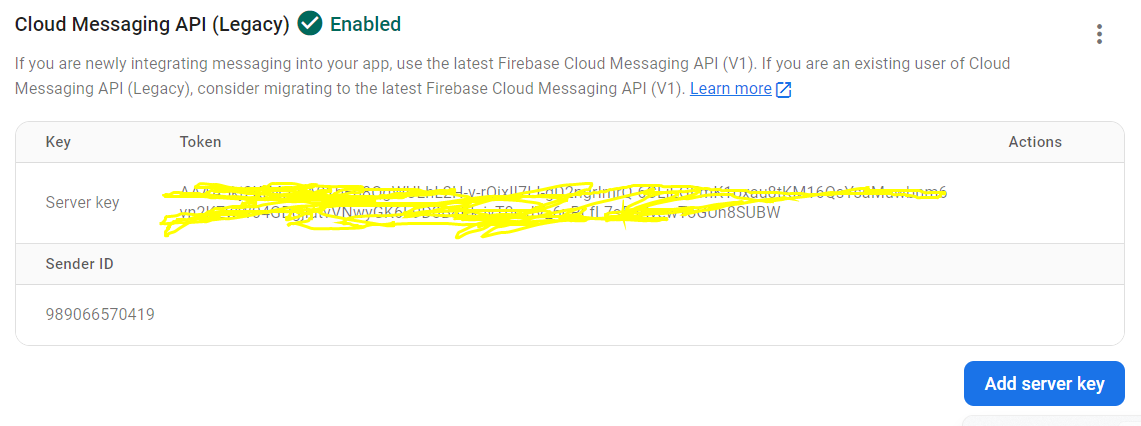
2:Creating Auth() login UI
After creating all the things now we good to go with .project after that if you have any login form then you may skip this else .....
Code for creating UI:Copy
composer require laravel/ui
php artisan ui bootstrap --auth
npm install
npm run dev
3:Creating Blade file
Create a blade file resources/views/home.blade.php and copy the below code
code for blade file : Copy
@extends('layouts.app')
@section('content')
<div class="container">
<div class="row justify-content-center">
<div class="col-md-8">
<button onclick="startFCM()" class="btn btn-danger btn-flat">Allow notification
</button>
<div class="card mt-3">
<div class="card-body">
@if (session('status'))
<div class="alert alert-success" role="alert">
{{ session('status') }}
</div>
@endif
<form action="{{ route('send.web-notification') }}" method="POST">
@csrf
<div class="form-group">
<label>Message Title</label>
<input type="text" class="form-control" name="title">
</div>
<div class="form-group">
<label>Message Body</label>
<textarea class="form-control" name="body"></textarea>
</div>
<button type="submit" class="btn btn-success btn-block">Send Notification</button>
</form>
</div>
</div>
</div>
</div>
</div>
<!-- The core Firebase JS SDK is always required and must be listed first -->
<script src="https://code.jquery.com/jquery-3.7.0.min.js" crossorigin="anonymous"></script>
<script src="https://www.gstatic.com/firebasejs/8.3.2/firebase.js"></script>
<script src="https://www.gstatic.com/firebasejs/8.10.1/firebase-messaging.js"></script>
<script>
var firebaseConfig = {
apiKey: '',
authDomain: '',
databaseURL: '',
projectId: '',
storageBucket: '',
messagingSenderId: '',
appId: '',
measurementId: '',
};
firebase.initializeApp(firebaseConfig);
const messaging = firebase.messaging();
function startFCM() {
messaging
.requestPermission()
.then(function() {
return messaging.getToken()
})
.then(function(response) {
$.ajaxSetup({
headers: {
'X-CSRF-TOKEN': '{{ csrf_token() }}'
}
});
$.ajax({
url: '{{ route("store.token") }}',
type: 'POST',
data: {
token: response
},
dataType: 'JSON',
success: function(response) {
alert('Token stored.');
},
error: function(error) {
alert(error);
},
});
}).catch(function(error) {
alert(error);
});
}
messaging.onMessage(function(payload) {
console.log(payload);
});
console.log(payload.notification.body);
const title = payload.notification.title;
const options = {
body: payload.notification.body,
icon: payload.notification.icon,
};
new Notification(title, options);
});
</script>
@endsection
4:Creating public/firebase-messaging-sw.js
Now create a file inside public folder public/firebase-messaging-sw.js as to google console verify application and also for handling notification at background
code for firebase-messaging-sw.js :Copy
// Give the service worker access to Firebase Messaging.
// Note that you can only use Firebase Messaging here. Other Firebase libraries
// are not available in the service worker.importScripts('https://www.gstatic.com/firebasejs/7.23.0/firebase-app.js');
importScripts('https://www.gstatic.com/firebasejs/8.3.2/firebase-app.js');
importScripts('https://www.gstatic.com/firebasejs/8.3.2/firebase-messaging.js');
/*
Initialize the Firebase app in the service worker by passing in the messagingSenderId.
*/
firebase.initializeApp({
apiKey: '',
authDomain: '',
databaseURL: '',
projectId: '',
storageBucket: '',
messagingSenderId: '',
appId: '',
measurementId: '',
});
// Retrieve an instance of Firebase Messaging so that it can handle background
// messages.
const messaging = firebase.messaging();
messaging.setBackgroundMessageHandler(function (payload) {
console.log("Message received.", payload);
const title = "Hello world is awesome";
const options = {
body: "Your notificaiton message .",
icon: "/firebase-logo.png",
};
return self.registration.showNotification(
title,
options,
);
});
5:Creating WebNotificationController
Create a WebNotificationController and paste the below code
code for controller: Copy
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\User;
class WebNotificationController extends Controller
{
public function index()
{
return view('home');
}
public function storeToken(Request $request)
{
auth()->user()->update(['device_key'=>$request->token]);
return response()->json(['Token successfully stored.']);
}
public function sendWebNotification(Request $request)
{
$url = 'https://fcm.googleapis.com/fcm/send';
$FcmToken = User::whereNotNull('device_key')->pluck('device_key')->all();
$serverKey = '';
$data = [
"registration_ids" => $FcmToken,
"notification" => [
"title" => $request->title,
"body" => $request->body,
]
];
$encodedData = json_encode($data);
$headers = [
'Authorization:key=' . $serverKey,
'Content-Type: application/json',
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 0);
curl_setopt($ch, CURLOPT_HTTP_VERSION, CURL_HTTP_VERSION_1_1);
// Disabling SSL Certificate support temporarly
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_POSTFIELDS, $encodedData);
// Execute post
$result = curl_exec($ch);
if ($result === FALSE) {
die('Curl failed: ' . curl_error($ch));
}
// Close connection
curl_close($ch);
// FCM response
print_r($result);
}
}
And also in web.php
code for web.php Copy
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\WebNotificationController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
*/
Route::get('/', function () {
return view('welcome');
});
Auth::routes();
Route::get('/home', [WebNotificationController::class, 'index'])->name('push-notificaiton');
Route::post('/store-token', [WebNotificationController::class, 'storeToken'])->name('store.token');
Route::post('/send-web-notification', [WebNotificationController::class, 'sendWebNotification'])->name('send.web-notification');
6:Sending Notification
Now start the project and send notification
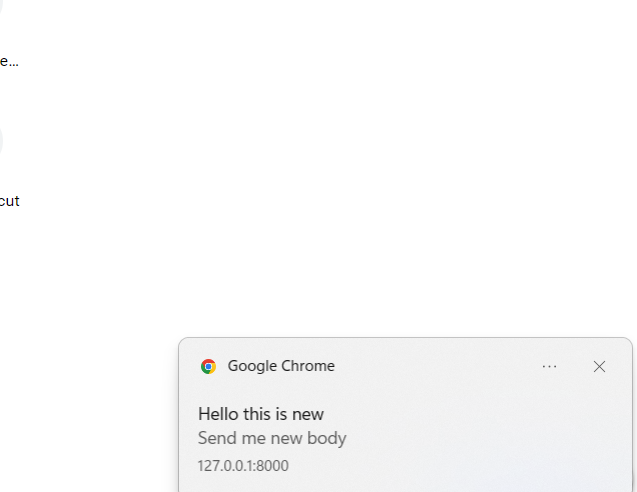
0 Comments (Please let us know your query)