Table of Contents
1:Introduction to roles
In many cases using laravel how could you define user's role like if my user has role customer then u may define is_role or role by column name in users table.
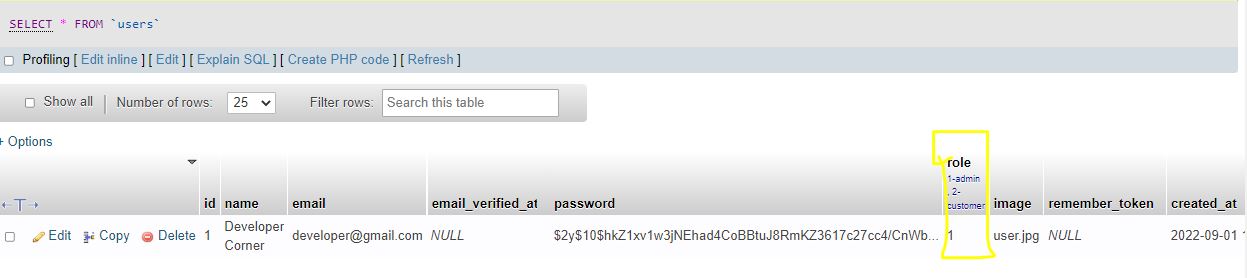
This works when your user has only single role but what if user has many roles like a user is a admin as well as customer , the option we have is to create a new user , but we have another option to attach many roles to a single user. we will make a function to attach different roles and call a function to get all users for role customer , in this example we will use Customer role.
2:Creating model and migration name Role
We will create a Model name Role and a migration file for creating table in database , in CMD or TERMINAL hit the folloeing command
command:Copy
php artisan make:model Role -m
This wiil create app/Models/Role.php and database/migrations/your timespan_create_roles_table.php files.
Inside database/migrations/your timespan_create_roles_table.php replace the following code
code:Copy
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('roles', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('slug');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('roles');
}
};
3:Run migration
After that now will create pivot table named user_roles where we use user_id and role_id as foreign key as following.
command:Copy
php artisan make:migration create_user_roles_table
Inside database/migrations/your timespan_create_user_roles_table.php replace the following code , as shown we are using foreign keys user_id and role_id from refrence users and roles as pivot table , as shown we are inializing user_id and role_id as unsignedBigInteger and after we creating refrence of these keys user_id on users and role_id on roles.
migration code:.Copy
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('user_roles', function (Blueprint $table) {
$table->id();
$table->unsignedBigInteger('user_id');
$table->unsignedBigInteger('role_id');
$table->foreign('user_id')->references('id')->on('users')->onDelete('cascade');
$table->foreign('role_id')->references('id')->on('roles')->onDelete('cascade');
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('user_roles');
}
};
Now run the followig command in your CMD and TERMINAL for creating roles and user_roles table
command: Copy
php artisan migrate
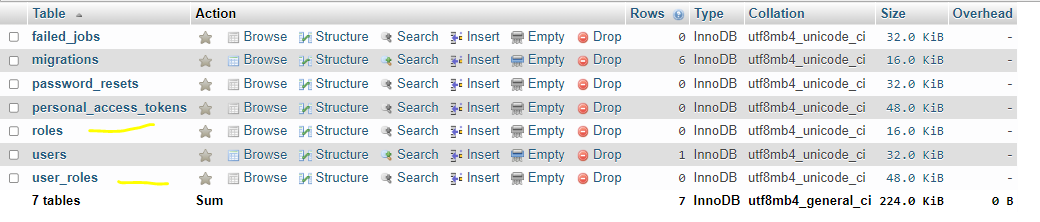
In user_roles Pivot table
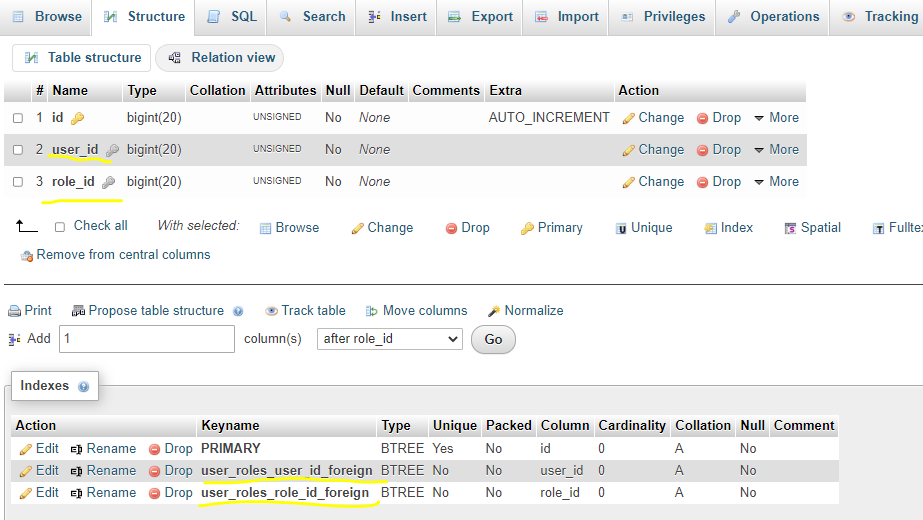
4:Creating belongsToMany relation in User model
Inside app/Models/User.php we are defining roles() and hasRole() where roles() function is used to get the roles of a particular user from user_roles table and hasRole() function is used whether the user has a perticular role or not paste the following code
code for model: Copy
<?php
namespace App\Models;
// use Illuminate\Contracts\Auth\MustVerifyEmail;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
use Laravel\Sanctum\HasApiTokens;
use Illuminate\Database\Eloquent\Casts\Attribute;
use URL;
class User extends Authenticatable
{
use HasApiTokens, HasFactory, Notifiable;
/**
* The attributes that are mass assignable.
*
* @var array<int, string>
*/
protected $fillable = [
'name',
'email',
'password',
];
/**
* The attributes that should be hidden for serialization.
*
* @var array<int, string>
*/
protected $hidden = [
'password',
'remember_token',
];
/**
* The attributes that should be cast.
*
* @var array<string, string>
*/
protected $casts = [
'email_verified_at' => 'datetime',
];
public function roles()
{
return $this->belongsToMany(Role::class,'user_roles');
}
public function hasRole(... $roles )
{
foreach ($roles as $role) {
if ($this->roles->contains('slug', $role)) {
return true;
}
}
return false;
}
}
5:Creating customer role
Now we had creted Role model along with migration , so now we will create Customer role and after will create user Custtomer and attach the role.
In web.php file Copy
use App\Models\Role;
Route::get('/createRole', function () {
$role = new Role();
$role->name = 'Customer';
$role->slug = 'customer';
$role->save();
});
6:Creating user and attach customer role
This wiil create a new role name Customer in database table roles.
After that now will create a customer so use any url along with controller to create a user after creating user we will assign customer role to user by using attach , as it will attach the role customer from model function belongToMany.
code:Copy
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\User;
use App\Models\Role;
use Hash;
use Auth;
class DeveloperCorner extends Controller
{
public function createCustomer()
{
$user = new User();
$user->name = 'Developer';
$user->password = Hash::make('1234');
$user->save();
$customer = Role::where('slug','customer')->first();
$user->roles()->attach($customer);
}
}
now your user_roles look something like this , as this is the pivot table where we use user_id and role_id as foreign keys from users and roles.
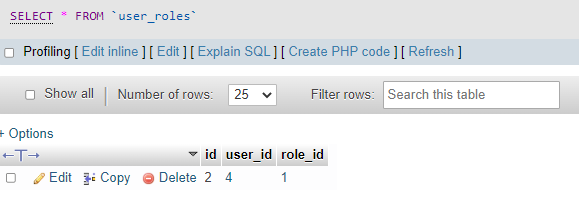
7:Get all customers users
Now we will get al the list of users having role Customer by getCustomer() , it will return all the users with role customer .
code:.Copy
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\User;
use App\Models\Role;
use Hash;
use Auth;
class DeveloperCorner extends Controller
{
public function createCustomer()
{
$user = new User();
$user->name = 'Developer';
$user->password = Hash::make('1234');
$user->save();
$customer = Role::where('slug','customer')->first();
$user->roles()->attach($customer);
}
public function getCustomer()
{
$role = 'customer';
$customer = User::whereHas('roles', function ($q) use ($role) {
$q->where('slug', $role);
})->get();
echo"<pre>";print_r($customer);
}
}
8:Middleware for customer role
And to use the condition to check the role of the user in middleware or in any other file use this
code for middleware:Copy
public function checkCustomer()
{
if(Auth::User()->hasRole('customer')){
}
}
0 Comments (Please let us know your query)