Table of Contents
1:What are traits ?
Traits are basically a peice of code where we reuse wherever we need it , like we need to save image in different controllers so what we do , we actually use move command and save the image , whcih results in repetive same code then we use traits. Hence traits are like objects where you can't direct call them , basically they are public protected functions which are mostly call by inside a function .
Here we are consider an example of api response.
2:Creating traits
For creating trait create a folder manually , traits inside app like this app/Traits and inside this traits make a file ApiResponse.php
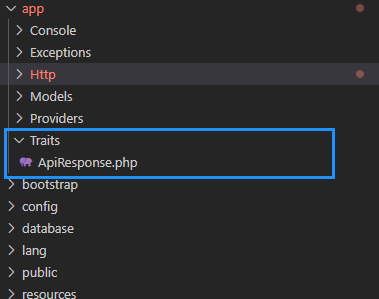
As shown below we are using an API response for success() and error() method which sends the response in json format by calling them from controller , paste the following code in app/Traits/ ApiResponse.php
code for traits Copy
<?php
namespace App\Traits;
use Carbon\Carbon;
/*
|--------------------------------------------------------------------------
| Api Responser Trait
|--------------------------------------------------------------------------
|
| This trait will be used for any response we sent to clients.
|
*/
trait ApiResponse
{
/**
* Return a success JSON response.
*
* @param array|string $data
* @param string $message
* @param int|null $code
* @return \Illuminate\Http\JsonResponse
*/
protected function success($data, string $message = null, int $code = 200)
{
return response()->json([
'status' => 'Success',
'message' => $message,
'data' => $data
], $code);
}
/**
* Return an error JSON response.
*
* @param string $message
* @param int $code
* @param array|string|null $data
* @return \Illuminate\Http\JsonResponse
*/
protected function error(string $message = null, int $code, $data = null)
{
return response()->json([
'status' => 'Error',
'message' => $message,
'data' => $data
], $code);
}
}
3:Calling traits
As shown in controller just initialize the traits namespace and used use ApiResponse for using traits in class
code for controller Copy
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\User;
use App\Models\Role;
use Hash;
use App\Traits\ApiResponse;
use Auth;
class DeveloperCorner extends Controller
{
use ApiResponse;
public function TestTraits()
{
$data = 'Developer Corner';
$message = 'Welcome to Developer Corner';
return $this->success($data,$message);
}
}
4:Result
I called this function by using TestTraits route through GET method
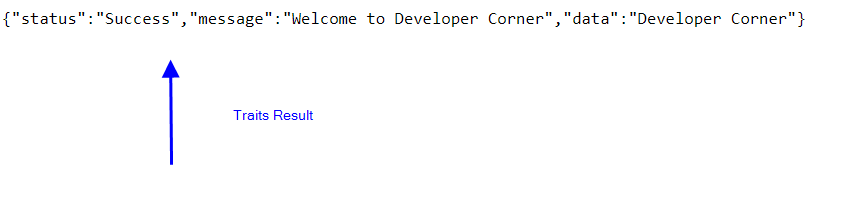
Now you may use and make flexible traits and feel free
0 Comments (Please let us know your query)